|
影片
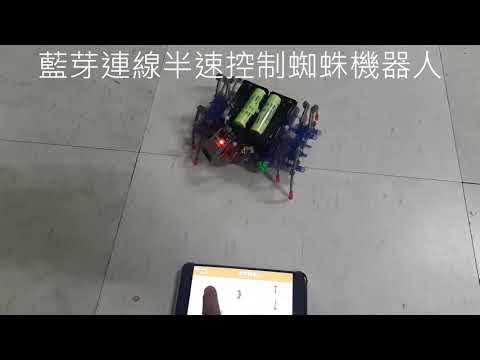
ESP32 控制 TB6612FNG 直流馬達驅動∕控制板 請看這篇
使用Android手機如何用Arduino藍芽連線ESP32控制蜘蛛機器人
需要使用雙電源
如果使用單電源,電流會被馬達抽走
ESP32晶片電流不足會無法正常運作
材料:
18650電池*2
ESP32*1
TB6612FNG*1
蜘蛛機器人*2
AUDINO 程式碼
- // Include necessary libraries
- #include <BLEDevice.h>
- #include <BLEServer.h>
- #include <BLEUtils.h>
-
- // 定義 UUIDs (注意要與App Inventor內容對應)
- #define SERVICE_UUID "C6FBDD3C-7123-4C9E-86AB-005F1A7EDA01"
- #define CHARACTERISTIC_UUID_RX "B88E098B-E464-4B54-B827-79EB2B150A9F"
- #define CHARACTERISTIC_UUID_TX "D769FACF-A4DA-47BA-9253-65359EE480FB"
-
- String BLE_Code;
- BLECharacteristic *pCharacteristic;
- bool deviceConnected = false;
- int directState = 0; // 動作
- int speed_c = 192;
- // PWM, INA, INB 與LED接腳變數
- int PWMA = 13;
- int INA1 = 12;
- int INA2 = 14;
- int STBY = 27;
- int INB1 = 25;
- int INB2 = 26;
- int PWMB = 33;
- const int ledPin = 2;
- const int freq = 10000;
- const int resolution = 8;
-
- // 設定 callbacks onConnect & onDisconnect函數
- class MyServerCallbacks: public BLEServerCallbacks {
- void onConnect(BLEServer* pServer) {
- deviceConnected = true;
- };
- void onDisconnect(BLEServer* pServer) {
- deviceConnected = false;
- }
- };
-
- // 設定 callback function 當收到新的資訊 (from the Android application)
- class MyCallbacks: public BLECharacteristicCallbacks {
- void onWrite(BLECharacteristic *pCharacteristic) {
- std::string rxValue = pCharacteristic->getValue();
- BLE_Code="";
- if(rxValue.length() > 0) {
- Serial.print("接收資料為 : ");
- for(int i = 0; i < rxValue.length(); i++) {
- BLE_Code+=rxValue[i];
- Serial.print(rxValue[i]);
- }
- Serial.println();
- BLE_Code.toUpperCase();
- Serial.println(BLE_Code);
- if(BLE_Code.indexOf("GO")!=-1)
- {
- Serial.println("GO");
- directState=1;
- } else if(BLE_Code.indexOf("BACK")!=-1) {
- Serial.println("BACK");
- directState=2;
- } else if(BLE_Code.indexOf("LEFT")!=-1) {
- Serial.println("LEFT");
- directState=3;
- } else if(BLE_Code.indexOf("RIGHT")!=-1) {
- Serial.println("RIGHT");
- directState=4;
- } else if(BLE_Code.indexOf("RELEASE")!=-1) {
- Serial.println("RELEASE");
- directState=5;
- }
- if(BLE_Code.indexOf("192")!=-1)
- {
- speed_c=192;
- } else if(BLE_Code.indexOf("255")!=-1) {
- speed_c=255;
- }
- }
- }
- };
-
- void setup() {
- Serial.begin(115200);
- pinMode(ledPin, OUTPUT); //設定腳位為輸出
- pinMode(INA1,OUTPUT);
- pinMode(INA2,OUTPUT);
- pinMode(PWMA,OUTPUT);
- pinMode(STBY,OUTPUT);
- pinMode(INB1,OUTPUT);
- pinMode(INB2,OUTPUT);
- pinMode(PWMB,OUTPUT);
- //digital output test
- digitalWrite(INA1,HIGH); //設定腳位HIGH LOW
- digitalWrite(INA2,LOW);
- digitalWrite(PWMA,LOW);
- digitalWrite(STBY,HIGH);
- digitalWrite(INB1,HIGH);
- digitalWrite(INB2,LOW);
- digitalWrite(PWMB,LOW);
- delay(1000);
- //analog output(PWM) test 設定LED Channel PWM 頻率
- ledcSetup(0, freq, resolution);
- ledcSetup(1, freq, resolution);
- ledcSetup(2, freq, resolution);
- ledcSetup(3, freq, resolution);
- ledcSetup(4, freq, resolution);
- ledcSetup(5, freq, resolution);
- ledcSetup(6, freq, resolution);
- //設定腳位Channel
- ledcAttachPin(INA1, 0);
- ledcAttachPin(INA2, 1);
- ledcAttachPin(PWMA, 2);
- ledcAttachPin(STBY, 3);
- ledcAttachPin(INB1, 4);
- ledcAttachPin(INB2, 5);
- ledcAttachPin(PWMB, 6);
-
- // 建立BLE Device
- BLEDevice::init("ESP32_WeMos1");
-
- // 建立BLE Server
- BLEServer *pServer = BLEDevice::createServer();
- pServer->setCallbacks(new MyServerCallbacks());
-
- // 建立BLE Service
- BLEService *pService = pServer->createService(SERVICE_UUID);
-
- // 建立BLE Characteristic
- pCharacteristic = pService->createCharacteristic(
- CHARACTERISTIC_UUID_TX,
- BLECharacteristic::PROPERTY_NOTIFY);
- // pCharacteristic->addDescriptor(new BLE2902());
- BLECharacteristic *pCharacteristic = pService->createCharacteristic(
- CHARACTERISTIC_UUID_RX,
- BLECharacteristic::PROPERTY_WRITE);
- pCharacteristic->setCallbacks(new MyCallbacks());
-
- // 開始(起)service
- pService->start();
-
- // 開始(起)advertising
- pServer->getAdvertising()->start();
- Serial.println("等待BLE手機連線....");
-
- digitalWrite(ledPin,LOW);
- delay(500);
- digitalWrite(ledPin,HIGH);
- delay(500);
- digitalWrite(ledPin,LOW);
- }
-
- void loop() {
- // Check received message and control output accordingly
- if (directState==1){
- move(speed_c, 1); // full speed, go
- Serial.println("前進");
- } else if(directState==2) {
- move(speed_c, 2); // full speed, down
- Serial.println("後退");
- } else if(directState==3) {
- move(speed_c, 3); // full speed, left
- Serial.println("左轉");
- } else if(directState==4) {
- move(speed_c, 4); // full speed, right
- Serial.println("右轉");
- } else if(directState==5) {
- stop(); //stop
- Serial.println("stop");
- }
- delay(100); //go for 1 second
- }
- void move( int speed, int direction){
- //Move specific motor at speed and direction
- //speed: 0 is off, and 255 is full speed
- //direction: 0 clockwise, 1 counter-clockwise
- ledcWrite(3, 255); //STBY disable standby
- Serial.println(direction);
- if(direction == 1){
- //設定馬達1為正轉
- ledcWrite(0, 255); //INA1
- ledcWrite(1, 0); //INA2
- ledcWrite(2, speed); //PWMA
- //設定馬達2為正轉
- ledcWrite(4, 255); //INB1
- ledcWrite(5, 0); //INB2
- ledcWrite(6, speed); //PWMB
- digitalWrite(ledPin,HIGH);
- delay(500);
- digitalWrite(ledPin,LOW);
- } else if (direction == 2){
- //設定馬達1為反轉
- ledcWrite(0, 0); //INA1
- ledcWrite(1, 255); //INA2
- ledcWrite(2, speed); //PWMA
- //設定馬達2為反轉
- ledcWrite(4, 0); //INB1
- ledcWrite(5, 255); //INB2
- ledcWrite(6, speed); //PWMB
- digitalWrite(ledPin,HIGH);
- delay(500);
- digitalWrite(ledPin,LOW);
- } else if (direction == 3){
- //設定馬達1為正轉
- ledcWrite(0, 255); //INA1
- ledcWrite(1, 0); //INA2
- ledcWrite(2, speed); //PWMA
- //設定馬達2為反轉
- ledcWrite(4, 0); //INB1
- ledcWrite(5, 255); //INB2
- ledcWrite(6, speed); //PWMB
- digitalWrite(ledPin,HIGH);
- delay(500);
- digitalWrite(ledPin,LOW);
- } else if (direction == 4){
- //設定馬達1為反轉
- ledcWrite(0, 0); //INA1
- ledcWrite(1, 255); //INA2
- ledcWrite(2, speed); //PWMA
- //設定馬達2為正轉
- ledcWrite(4, 255); //INB1
- ledcWrite(5, 0); //INB2
- ledcWrite(6, speed); //PWMB
- digitalWrite(ledPin,HIGH);
- delay(500);
- digitalWrite(ledPin,LOW);
- }
- Serial.println(speed);
- }
- void stop(){
- //enable standby
- ledcWrite(3, 0); //STBY enable standby
- //Serial.println("stop");
- }
複製代碼
ARDUINO 程式
ESP32_BLE_car_20200713.rar
(2.12 KB, 下載次數: 2, 售價: 10 金T幣)
ANDROID APK檔案
BluetoothControl_2020071301.rar
(1.95 MB, 下載次數: 6, 售價: 10 金T幣)
文章出處:網頁設計,網站架設 ,網路行銷,網頁優化,SEO - NetYea 網頁設計
|
|